10 Essential Secure Coding Practices Every Developer Should Follow
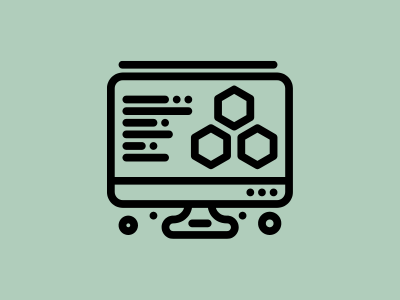
In today’s digital landscape, where cyber threats are increasingly sophisticated, ensuring the security of software applications is paramount. Secure coding practices play a crucial role in fortifying software against vulnerabilities and attacks. By adhering to established security principles, developers can mitigate risks and safeguard sensitive data. In this comprehensive guide, we’ll explore a secure coding practices checklist, detailing ten essential practices that every developer should prioritize to enhance the security of their software.
Table of Contents
Input Validation:
Input validation is the first line of defense against malicious input. Developers should validate all user inputs to ensure they conform to expected formats, lengths, and ranges. By sanitizing inputs, developers can prevent injection attacks such as SQL injection, cross-site scripting (XSS), and command injection.
Example:
// Validate email input
function isValidEmail(email) {
return /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(email);
}
Output Encoding:
Encoding output data prevents script injection attacks and ensures that user-supplied content is displayed safely. Developers should encode special characters before rendering data to prevent XSS vulnerabilities.
Example:
// Encode HTML output
function encodeHTML(input) {
return input.replace(/&/g, "&amp;").replace(/</g, "&lt;").replace(/>/g, "&gt;").replace(/"/g, "&quot;").replace(/'/g, "&#x27;").replace(/\//g, "&#x2F;");
}
Authentication and Authorization:
Implement strong authentication mechanisms to verify the identity of users. Additionally, enforce proper authorization checks to ensure that authenticated users have appropriate access rights to resources and functionalities.
Example:
// Authenticate user
function authenticate(username, password) {
// Check credentials against database
// Return true if authenticated, false otherwise
}
// Authorize user
function authorize(user, resource) {
// Check user's permissions for the resource
// Return true if authorized, false otherwise
}
Data Encryption:
Encrypt sensitive data both at rest and in transit to protect it from unauthorized access. Utilize strong encryption algorithms and secure key management practices to safeguard confidential information.
Example:
// Encrypt data
function encryptData(data, key) {
// Implement encryption algorithm
// Return encrypted data
}
// Decrypt data
function decryptData(encryptedData, key) {
// Implement decryption algorithm
// Return decrypted data
}
Error Handling:
Proper error handling can prevent information leakage and help developers identify and address security flaws. Implement customized error messages and avoid revealing sensitive system details to users.
Example:
try {
// Risky operation
} catch (error) {
// Log error without exposing sensitive details
console.error("An error occurred. Please try again later.");
}
Session Management:
Secure session management is essential to prevent session hijacking and fixation attacks. Use secure cookies, enforce session timeouts, and regenerate session identifiers after authentication.
Example:
// Set secure session cookie
res.cookie('sessionID', sessionId, { secure: true, httpOnly: true, sameSite: 'strict' });
Secure Configuration:
Ensure that default configurations are secure and minimize the attack surface by disabling unnecessary services and features. Regularly update software dependencies and libraries to patch known vulnerabilities.
Least Privilege Principle:
Adhere to the principle of least privilege by granting users only the permissions necessary to perform their tasks. Limit access to sensitive resources and functionalities to mitigate the impact of potential security breaches.
Secure Communication:
Implement secure communication protocols such as HTTPS to encrypt data transmitted between clients and servers. Validate server certificates to prevent man-in-the-middle attacks and ensure the integrity of communications.
Security Testing and Review:
Regularly conduct security testing, code reviews, and vulnerability assessments to identify and remediate security weaknesses. Utilize automated tools and manual inspection to comprehensively assess the security posture of the software.
In conclusion, adopting secure coding practices is imperative for building resilient and trustworthy software applications. By integrating these ten essential practices into their development processes, developers can bolster the security of their software and mitigate the risk of cyber threats. Prioritizing security from the outset is essential for safeguarding sensitive data and maintaining user trust in an increasingly interconnected digital ecosystem.